νμ΄μ¬μμ μ€νμ λ©μλ(λλ λ§€μ§ λ©μλ)λ μ΄μ€ μΈλμ€μ½μ΄λ‘ λλ¬μΈμΈ μ΄λ¦μ κ°μ§λ©°, κ°μ²΄μ νΉμ λμμ μ μνλ λ° μ¬μ©λ©λλ€. μ΄λ¬ν λ©μλλ€μ ν΄λμ€μ μ μλλ©°, λ΄μ₯ ν¨μλ μ°μ°μ λ±κ³Ό μνΈ μμ©νλλ‘ μ€κ³λμ΄ μμ΅λλ€. λ€μμ λͺ κ°μ§ μ£Όμ μ€νμ λ©μλμ μ’ λ₯μ μμμ λλ€.
1. __init__(self, ...): κ°μ²΄κ° μμ±λ λ νΈμΆλλ λ©μλλ‘, μ΄κΈ°νλ₯Ό λ΄λΉν©λλ€.
class MyClass:
def __init__(self, x):
self.x = x
obj = MyClass(10)
2. __str__(self), __repr__(self): κ°μ²΄λ₯Ό λ¬Έμμ΄λ‘ νννλλ° μ¬μ©λλ λ©μλμ λλ€.
class MyClass:
def __init__(self, x):
self.x = x
def __str__(self):
return "This is a MyClass object."
def __repr__(self):
return f"MyClass({self.x})"
obj = MyClass(10)
print(obj)
print(repr(obj))
# This is a MyClass object.
# MyClass(10)
3. __len__(self): κ°μ²΄μ κΈΈμ΄λ₯Ό λ°ννλ λ©μλλ‘, λ΄μ₯ ν¨μ len()κ³Ό ν¨κ» μ¬μ©λ©λλ€.
class MyList:
def __init__(self, items):
self.items = items
def __len__(self):
return len(self.items)
my_list = MyList([1, 2, 3, 4])
print(len(my_list))
4. __add__(self, other): κ°μ²΄ κ°μ λ§μ μ°μ°μ μ μνλ λ©μλλ‘, μ°μ°μ + μ ν¨κ» μ¬μ©λ©λλ€.
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Point(self.x + other.x, self.y + other.y)
p1 = Point(1, 2)
p2 = Point(3, 4)
p3 = p1 + p2
5. __getitem__(self, key), __setitem__(self, key, value): μΈλ±μ± λ° ν λΉμ μ μνλ λ©μλμ λλ€.
class MyList:
def __init__(self, items):
self.items = items
def __getitem__(self, index):
return self.items[index]
def __setitem__(self, index, value):
self.items[index] = value
my_list = MyList([1, 2, 3, 4])
print(my_list[2])
my_list[2] = 10
6. __eq__(self, other): λ κ°μ²΄μ λλ±μ±μ κ²μ¬νλ λ©μλμ λλ€. == μ°μ°μμ ν¨κ» μ¬μ©λ©λλ€.
class MyClass:
def __init__(self, x):
self.x = x
def __eq__(self, other):
return self.x == other.x
obj1 = MyClass(10)
obj2 = MyClass(10)
print(obj1 == obj2)
7. __lt__(self, other), __gt__(self, other): κ°μ²΄ κ°μ μμμ§(<), ν°μ§(>) λΉκ΅λ₯Ό μ μνλ λ©μλμ λλ€.
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __lt__(self, other):
return self.x < other.x and self.y < other.y
def __gt__(self, other):
return self.x > other.x and self.y > other.y
p1 = Point(1, 2)
p2 = Point(3, 4)
print(p1 < p2)
print(p1 > p2)
8. __enter__(self), __exit__(self, exc_type, exc_value, traceback): 컨ν μ€νΈ κ΄λ¦¬λ₯Ό μν λ©μλλ‘, with λ¬Έκ³Ό ν¨κ» μ¬μ©λ©λλ€.
class MyContext:
def __enter__(self):
print("Entering the context")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Exiting the context")
with MyContext() as context:
print("Inside the context")
μ΄ λ©μλλ€μ ꡬννλ©΄ ν΄λΉ ν΄λμ€μ μΈμ€ν΄μ€λ₯Ό with λ¬Έμμ μ¬μ©ν μ μμΌλ©°, __exit__μμ μμΈ μ²λ¦¬ λ±μ μνν μ μμ΅λλ€.
9. __call__: κ°μ²΄λ₯Ό ν¨μμ²λΌ νΈμΆν μ μλλ‘ νλ λ©μλμ λλ€. μ¦, ν΄λΉ ν΄λμ€μ μΈμ€ν΄μ€λ₯Ό λ§μΉ ν¨μμ²λΌ νΈμΆν μ μκ² λ©λλ€. μ΄ λ©μλλ₯Ό μ¬μ©νλ©΄ κ°μ²΄κ° νΈμΆλ λ νΉμ λμμ μ μν μ μμ΅λλ€.
class CallableClass:
def __call__(self, *args, **kwargs):
print("Object is being called with arguments:", args)
return sum(args)
# κ°μ²΄λ₯Ό μμ±
callable_obj = CallableClass()
# κ°μ²΄λ₯Ό ν¨μμ²λΌ νΈμΆ
result = callable_obj(1, 2, 3)
print("Result of the call:", result)
μμ μμ μμ `CallableClass`λ `__call__` λ©μλλ₯Ό μ μνκ³ μμ΅λλ€. μ΄ λ©μλλ κ°μ²΄κ° νΈμΆλ λ μ€νλλ©°, νΈμΆλ μΈμλ€μ μΆλ ₯νκ³ μΈμλ€μ ν©μ λ°νν©λλ€.
`__call__`μ μ¬μ©νλ©΄ κ°μ²΄λ₯Ό μΌλ°μ μΈ ν¨μμ²λΌ μ¬μ©ν μ μμ΄μ, ν¨μμ μ μ¬ν λμμ νλ κ°μ²΄λ₯Ό λ§λ€ μ μμ΅λλ€. μ΄κ²μ ν¨μλ₯Ό μΈμλ‘ λ°κ±°λ λ°ννλ λ±μ μν©μμ μ μ©ν μ μμ΅λλ€.
μ΄ μΈμλ λ€μν μ€νμ
λ©μλκ° μμΌλ©°, μ΄λ¬ν λ©μλλ€μ μ νμ©νλ©΄ κ°μ²΄μ λμμ 컀μ€ν°λ§μ΄μ§ν μ μμ΅λλ€. κ·Έλ μ§λ§ μ§κΈ μκ°ν΄λλ¦° μ€νμ
λ©μλμ νμ©λκ° 90νλ‘ μ΄μ λκΈ° λλ¬Έμ, μ΄ λ©μλλ€λ§ μ΅μν΄μ§λ©΄ μ½λλ₯Ό λ³Ό λ ν¬κ² λΆνΈν¨μ΄ μμΌμ€ κ²λλ€!
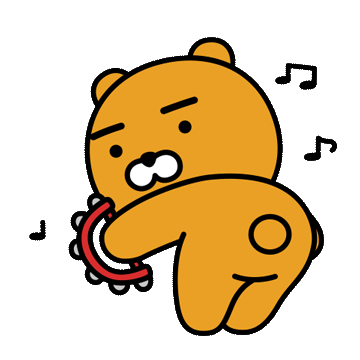
'νλ‘κ·Έλλ° μΈμ΄ > Python' μΉ΄ν κ³ λ¦¬μ λ€λ₯Έ κΈ
νμ΄μ¬ map, filter ν¨μ(with. iterator) (0) | 2023.12.17 |
---|---|
νμ΄μ¬ 리μ€νΈ μ»΄ν리ν¨μ , λμ λ리 μ»΄ν리ν¨μ μ λͺ¨λ κ² (0) | 2023.12.12 |
νμ΄μ¬ μμΈμ²λ¦¬(Exception)μ λͺ¨λ κ² (1) | 2023.12.10 |
νμ΄μ¬ λͺ¨λκ³Ό ν¨ν€μ§μ λͺ¨λ κ² (0) | 2023.12.09 |
νμ΄μ¬ μ μΆλ ₯(νμ€, νμΌ λ±)μ λͺ¨λ κ² (1) | 2023.12.05 |
λκΈ